템플릿 콜백 패턴으로 반복 코드 줄이기
같은 코드의 반복은 중복을 낳는다. 그리고 중복은 유지 보수를 어렵게 만든다. 따라서 반복을 최소화해야 한다.
이 글은 엑셀 파일 데이터를 가공하여 데이터베이스로 옮기거나 혹은 다른 파일 포맷으로 변경하려 할 때 나타날 수 있는 반복 코드와 템플릿/콜백Template/Callback 패턴을 사용하여 반복 코드를 줄여가는 과정을 소개한다.
반복 코드
엑셀 파일을 읽어 오는 코드를 작성한다고 가정해 보자.
Java로 엑셀 파일을 다룰 때 Apache POI(이하 POI)를 많이 사용한다. POI는 마이크로소프트 오피스 포맷(Word, PowerPoint, Excel) 문서를 읽고 쓸 수 있는 Java 라이브러리이다.[1]
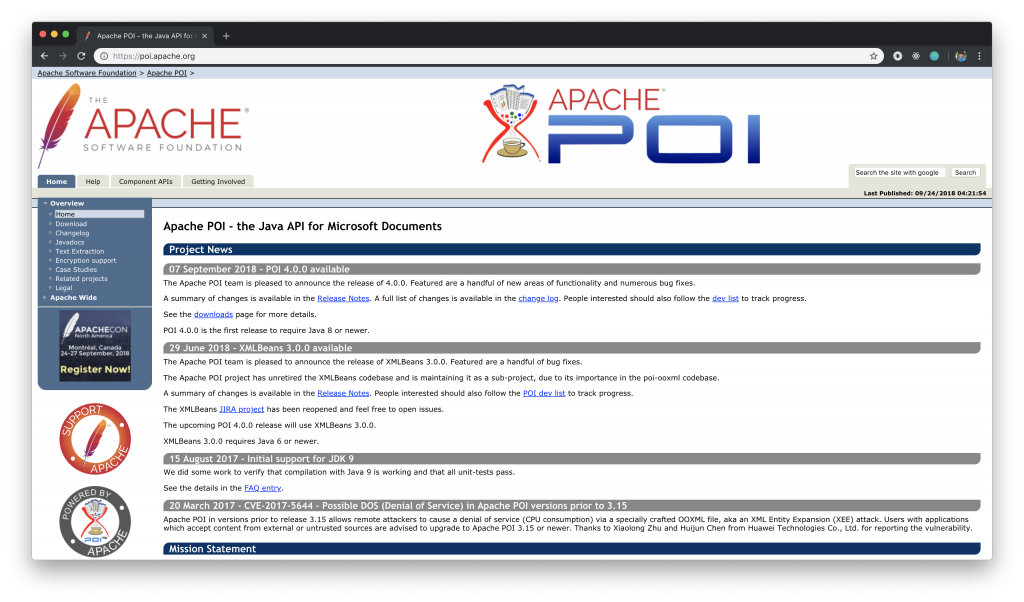
https://poi.apache.org/
POI 라이브러리를 사용하여 엑셀 파일을 읽어 오는 코드를 아래같이 작성할 수 있다.
Excel 클래스의 getRows() 메서드는 엑셀 파일 각 행을 읽어 Java Map(Key는 column index, Value는 cell 값)으로 변환하고 이를 List에 담아 반환한다.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
//... import org.apache.poi.ss.usermodel.*; import org.apache.poi.xssf.usermodel.XSSFWorkbook; public class Excel { private Workbook workbook; public Excel(File file) throws IOException { // 1. 파일을 workbook 객체로 생성 FileInputStream fis = new FileInputStream(file); this.workbook = new XSSFWorkbook(fis); } public List<Map<Integer, String>> getRows(String sheetName) { // 2. 엑셀 시트 조회 Sheet sheet = workbook.getSheet(sheetName); List<Map<Integer, String>> rows = new ArrayList<>(); final DataFormatter formatter = new DataFormatter(); // 3. 각 행 처리 for (Row row : sheet) { // 4. 각 행의 열을 Java Map으로 생성 Map<Integer, String> rowMap = new HashMap<>(); for (Cell cell : row) { String text = formatter.formatCellValue(cell); rowMap.put(cell.getColumnIndex(), text); } // 5. 행 List에 추가 rows.add(rowMap); } return rows; } } public class Client { public static void main(String[] args) throws IOException { final String filePath = getClass().getClassLoader().getResource("sample.xlsx").getPath(); Excel excel = new Excel(new File(filePath)); List<Map<Integer, String>> rows = excel.getRows("Sheet1"); System.out.println(rows); // [{0=1, 1=product a, 2=200}, {0=2, 1=product b, 2=300}, {0=3, 1=product c, 2=400}, {0=4, 1=product d, 2=110}, {0=5, 1=product e, 2=211}] } }
단순하게 엑셀 파일을 읽어오는 경우라면 위의 코드를 사용해도 무방하지만 문제 영역에 따라 엑셀 파일은 매우 다양하게 처리해야 한다.
- 첫 번째 행을 헤더로 사용하고 싶다.
- 셀 서식을 확인하여 특정 데이터 타입(예. Date, Integer 등)으로 사용하고 싶다.
- 엑셀 행을 Map 형태가 아닌 구체적인 클래스로 사용하고 싶다.
문제는 엑셀 행 처리만 다를 뿐 나머지 코드는 동일하기 때문에 별도의 클래스를 만들면 코드 반복이 일어날 수 있다는 것이다.
변하는 것과 변하지 않는 것
엑셀 파일을 Workbook으로 만들고 Sheet를 조회하여 모든 행을 순환하는 부분은 변하지 않는 것이다. 반면 변하는 것은 두 가지인데 '행 처리'와 '반환 데이터 타입'이다.
템플릿과 콜백
반복되는 코드를 제거하기 위해서 템플릿/콜백 개념을 도입해보자.
먼저 템플릿은 어떤 목적을 위해 미리 만들어둔 모양이 있는 틀을 가르키며, 콜백은 실행되는 것을 목적으로 다른 오브젝트의 메소드에 전달되는 오브젝트를 말한다. 파라미터로 전달되지만 값을 참조하기 위한 것이 아니라 특정 로직을 담은 메소드를 실행 시키기 위해 사용한다.[2]
Excel 클래스의 변하지 않는 것을 템플릿으로 만들고 변하는 부분은 콜백으로 만들어보자.
ExcelTemplate 클래스는 변하지 않는 부분을 담당하고 변하는 부분은 콜백으로써 ExcelRowMapper 인터페이스로 위임한다. 그리고 Client는 콜백으로 ExcelRowMapper 인터페이스를 구현한다.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41
public interface ExcelRowMapper { Map<Integer, String> mapRow(Row row); } public class ExcelTemplate { private Workbook workbook; public ExcelTemplate(File file) throws IOException { FileInputStream fis = new FileInputStream(file); this.workbook = new XSSFWorkbook(fis); } public List<Map<Integer, String>> getRows(String sheetName, ExcelRowMapper rowMapper) { Sheet sheet = workbook.getSheet(sheetName); List<Map<Integer, String>> rows = new ArrayList<>(); for (Row row : sheet) { Map<Integer, String> rowMap = rowMapper.mapRow(row); rows.add(rowMap); } return rows; } } public class Client { public static void main(String[] args) throws IOException { final String filePath = Client.class.getClassLoader().getResource("sample.xlsx").getPath(); ExcelTemplate excelTemplate = new ExcelTemplate(new File(filePath)); final DataFormatter formatter = new DataFormatter(); List<Map<Integer, String>> rows = excelTemplate.getRows("Sheet1", // 콜백 new ExcelRowMapper() { @Override public Map<Integer, String> mapRow(Row row) { Map<Integer, String> rowMap = new HashMap<>(); for (Cell cell : row) { String text = formatter.formatCellValue(cell); rowMap.put(cell.getColumnIndex(), text); } return rowMap; } }); System.out.println(rows); // [{0=1, 1=product a, 2=200}, {0=2, 1=product b, 2=300}, {0=3, 1=product c, 2=400}, {0=4, 1=product d, 2=110}, {0=5, 1=product e, 2=211}] } }
반환 데이터 타입은?
위의 코드에서 반환 데이터 타입은 Map이다. Java 제네릭스Generics[2]를 사용하여 Map이 아닌 Client가 원하는 타입으로 변경이 가능하다. 제네릭스로 반환 타입을 Client가 결정하도록 코드를 변경해보자.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50
public interface ExcelRowMapper<T> { T mapRow(Row row); } public class ExcelTemplate { private Workbook workbook; public ExcelTemplate(File file) throws IOException { FileInputStream fis = new FileInputStream(file); this.workbook = new XSSFWorkbook (fis); } public <T> List<T> getRows(String sheetName, ExcelRowMapper<T> rowMapper) { Sheet sheet = workbook.getSheet(sheetName); List<T> rows = new ArrayList<>(); for (Row row : sheet) { rows.add(rowMapper.mapRow(row)); } return rows; } } public class Client { public static void main(String[] args) throws IOException { final String filePath = Client.class.getClassLoader().getResource("sample.xlsx").getPath(); ExcelTemplate excelTemplate = new ExcelTemplate(new File(filePath)); List<Product> rows = excelTemplate.getRows("Sheet1", new ExcelRowMapper<Product>() { @Override public Product mapRow(Row row) { Product product = new Product(); for (Cell cell : row) { switch (cell.getColumnIndex()) { case 0 : product.setId(Double.valueOf(cell.getNumericCellValue()).intValue()); break; case 1 : product.setName(cell.getStringCellValue()); break; case 2 : product.setPrice(cell.getNumericCellValue()); break; } } return product; } }); } } public class Product { private int id; private String name; private double price; //... }
Java 8 이상을 사용하고 있다면
람다Lambda로 아래처럼 코드를 변경할 수 있다.
1 2 3 4 5 6 7 8 9 10
public class Client { public static void main(String[] args) throws IOException { // ... List<Product> rows = excelTemplate.getRows("Sheet1", row -> { //... return product; }); // ... } }